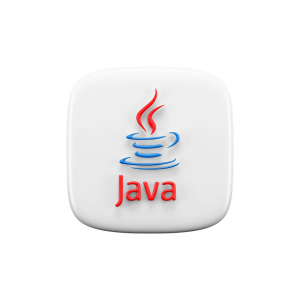
시작
유튜브에서 언어 활용도를 높이려면 이런것들을 자주 만들면 좋다고 해서 한 번 해볼까 한다.
가위, 바위, 보 출력 프로그램을 만들어서 intelliJ에서 사용해보기? 임의로 만드는거라 요구사항을 따로 없다.
풀이
import java.util.Scanner;
import java.util.Random;
public class Main {
public static void main(String[] args) {
String[] rps = {"가위", "바위", "보"};
Scanner scanner = new Scanner(System.in);
Random random = new Random();
System.out.println("가위, 바위, 보 중 하나를 입력해주세요.");
String userChoice = scanner.nextLine();
String computerChoice = rps[random.nextInt(rps.length)];
System.out.println("사용자: " + userChoice);
System.out.println("컴퓨터: " + computerChoice);
if (userChoice.equals(computerChoice)) {
System.out.println("비겼습니다.");
} else if ((userChoice.equals("가위") && computerChoice.equals("보")) ||
(userChoice.equals("바위") && computerChoice.equals("가위")) ||
(userChoice.equals("보") && computerChoice.equals("바위"))) {
System.out.println("당신이 이겼습니다.");
} else {
System.out.println("당신이 졌습니다.");
}
}
}
어... 거의 2시간은 걸린것 같다.
성공했다...ㅎㅎ;;
String userChoice = scanner.nextLine();
String computerChoice = rps[random.nextInt(rps.length)];
여기에 nextLine 이라는 메서드를 몰라서 계속 삽질하다가 구글링을 해서 끼워넣었다...😮💨
정리를 해보자면...
String[] rps = {"가위", "바위", "보"};
Scanner scanner = new Scanner(System.in);
Random random = new Random();
우선 문자열의 배열이기 때문에 String[] 을 사용했고 rock, scissor, paper라서 rps로 했다. rsp지만 어감이 rps가 더 좋아서 이렇게 설정했다. 그 다음 줄엔 입력값을 받아주어야 하기때문에 Scanner(System.in)를 넣어주었고 무작위 랜덤값을 꺼내야해서 random을 사용했다.
System.out.println("가위, 바위, 보 중 하나를 입력해주세요.");
String userChoice = scanner.nextLine();
String computerChoice = rps[random.nextInt(rps.length)];
입력값을 받아 넣긴 넣어야하는데 뭘 사용해야할지 몰라서 여기서 제일 시간을 많이 사용한 것 같다. String userChoice에 scanner.nextLine() 즉 사용자가 입력한 값을 고대로 useChoice에 저장해주기 때문에 String을 선택했고 3열에 computerChoice 객체에는 rps배열에서 무작위로 돌린? 저 셋 중에 하나가 나와야하기 때문에 rps.length를 선택했다.
별거 아니었는데 ... 참 오래도 고민했다.
마지막으로 제일 중요한 로직? 이라고 해도 될지 모르지만 if문인데
if (userChoice.equals(computerChoice)) {
System.out.println("비겼습니다.");
} else if ((userChoice.equals("가위") && computerChoice.equals("보")) ||
(userChoice.equals("바위") && computerChoice.equals("가위")) ||
(userChoice.equals("보") && computerChoice.equals("바위"))) {
System.out.println("당신이 이겼습니다.");
} else {
System.out.println("당신이 졌습니다.");
}
3가지 경우의 수가 있기 때문에 if, else if, else 가 전부 나오게 되었다. 비기는 경우는 userChoice값과 computerChoice값이 동일하게 나왔을 때만 생각하면 되기 때문에 좀 수월했는데 && or연산자?와 || 를 간만에 실사용해본 것 같다. 이기는 경우의 수는 가위, 바위, 보 각각 한번씩이기 때문에 3가지 조건만 넣으면 완성이 되었고 이외 경우는 졌다는 것 밖에 없기 때문에 else를 통해 졌다는 것을 출력해주었다.
그런데 문제가 하나 있다.
배열에 정해둔 문자열 이외에 작성을 해도 연산이 되버린다.
오늘 사고회로에 임계점에 도달했는데 이대로 가기엔 너무 아쉬웠다.
import java.util.Scanner;
import java.util.Random;
public class Main {
public static void main(String[] args) {
String[] rps = {"가위", "바위", "보"};
Scanner scanner = new Scanner(System.in);
Random random = new Random();
System.out.println("가위, 바위, 보 중 하나를 입력해주세요.");
String userChoice = scanner.nextLine();
while (!isValidChoice(userChoice)) {
System.out.println("잘못된 입력입니다. 가위, 바위, 보 중 하나를 다시 입력해주세요.");
userChoice = scanner.nextLine();
}
String computerChoice = rps[random.nextInt(rps.length)];
System.out.println("사용자: " + userChoice);
System.out.println("컴퓨터: " + computerChoice);
if (userChoice.equals(computerChoice)) {
System.out.println("비겼습니다.");
} else if ((userChoice.equals("가위") && computerChoice.equals("보")) ||
(userChoice.equals("바위") && computerChoice.equals("가위")) ||
(userChoice.equals("보") && computerChoice.equals("바위"))) {
System.out.println("당신이 이겼습니다.");
} else {
System.out.println("당신이 졌습니다.");
}
}
private static boolean isValidChoice(String choice) {
return choice.equals("가위") || choice.equals("바위") || choice.equals("보");
}
}
조건이 맞지않으면 코드가 실행도 되지 않게 하기위해선 while문은 참(True)일 경우에만 돌아가는 특성을 이용한다면 간단하게 해결된다.근데 조건 맞추는 함수를 몰라서 요건 GPT의 도움을 조금 받았다.
이럴 경우 지정한 문자열만 선택지에 넣을 수 있도록 하는 함수를 하나 만들어주어야 한다고 한다.
private static boolean isValidChoice(String choice) {
return choice.equals("가위") || choice.equals("바위") || choice.equals("보");
}
isValidChoice에 들어있는 여기 속에 있는 이 녀석들이 아니라면 할 수 없도록? 하면 되는데 ! 하나 붙이면 부정으로 바꿀 수 있어서 이 정도면 된다.
while (!isValidChoice(userChoice)) {
System.out.println("잘못된 입력입니다. 가위, 바위, 보 중 하나를 다시 입력해주세요.");
userChoice = scanner.nextLine();
}
입력값이 유효하지 않을 경우에는 computerChoice로 넘어가지 못하도록 userChoice와 computerChoice 사이에 배치했다.
선택하는 쪽이 한 곳이기 때문에 꽤 편하게 된듯 싶다?
잘 출력이 되는 모습이다. 강의만 따라 들어서 그런지 주도적으로 함수도 만들고 해서 필요에 따라 유연하게 사고해야 하는데 이게 잘 안된다. 답답하군...
마치며
자바 스프링을 제대로 공부한지 4개월쯤 되어가는데 이거 만드려니깐 심장이 쿵쾅쿵쾅했다...
다음엔 계산기까지 만들어봐야하는데 잘 할 수 있겠지? 사실 계산기는 어떤 문법을 써야할지 종이에 적어가며 또 고민을 할듯하다.
입력값 관련해서는 이것저것 신기한 것들을 참 많이 배워간다. 재밌으면서도 현타가 참 많이왔던 시간이었다😨😨
쓰임 있는 사람이 되기 위해 노력 중입니다.